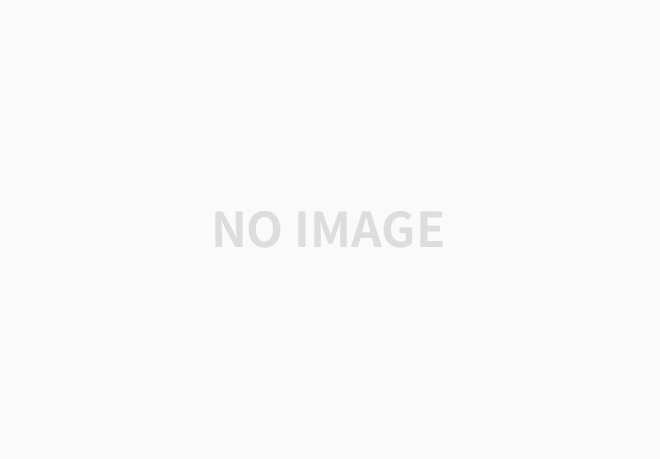
class SutdaCard{
//카드의 숫자.(1~10사이의 정수)
int num;
//광이면 true, 아니면 false
boolean isKwang;
}
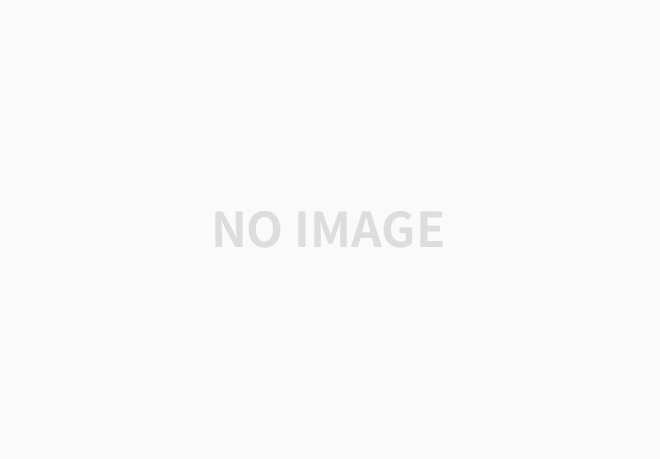
package objectprogm1;
public class Q6_2 {
public static void main(String[] args) {
SutdaCard card1 = new SutdaCard(3, false);
SutdaCard card2 = new SutdaCard();
System.out.println(card1.info());
System.out.println(card2.info());
}
}
class SutdaCard{
final static int MIN_NUM = 1;
final static int MAX_NUM = 10;
int num;
boolean isKwang;
SutdaCard(){
this(1, true);
}
SutdaCard(int num, boolean isKwang){
this.num = num;
this.isKwang = isKwang;
}
String info() {
return num + (isKwang? "K" : "");
/*
아래에서는 String.format() 사용
if(isKwang) {
return String.format("%dK",this.num);
}else {
return String.format("%d",this.num);
}
*/
}
}
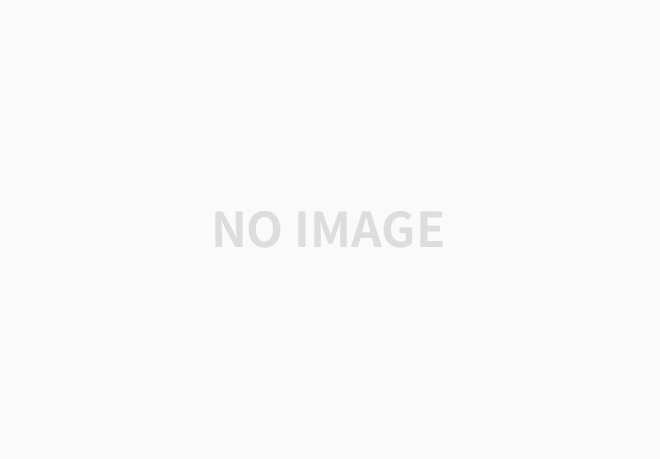
class Student{
String name;
int ban;
int no;
int kor;
int eng;
int math;
}
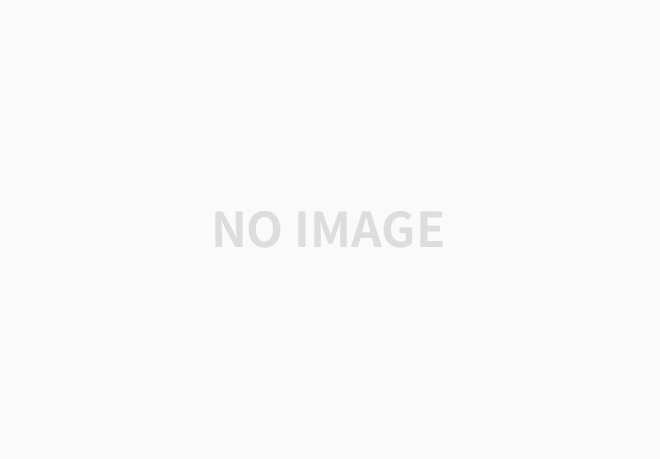
class Student{
String name;
int ban;
int no;
int kor;
int eng;
int math;
int getTotal() {
return kor+eng+math;
}
float getAverage() {
return (int)(getTotal()/3f*10+0.5)/10f;
}
}
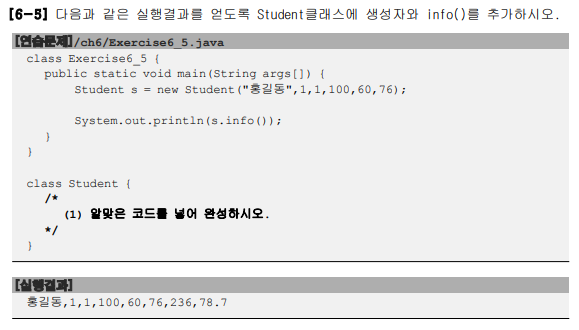
package objectprogm1;
public class Q6_5 {
public static void main(String[] args) {
Student s = new Student("홍길동",1,1,100,60,76);
System.out.println(s.info());
}
}
class Student{
String name;
int ban;
int no;
int kor;
int eng;
int math;
Student(){
this("아무나",0,0,100,100,100);
}
Student(String name, int ban, int no, int kor, int eng, int math){
this.name = name;
this.ban = ban;
this.no = no;
this.kor = kor;
this.eng = eng;
this.math = math;
}
String info() {
return String.format("%s,%d,%d,%d,%d,%d,%d,%.1f",this.name,this.ban,this.no,this.kor,this.eng,this.math,this.getTotal(),this.getAverage());
}
int getTotal() {
return kor+eng+math;
}
float getAverage() {
return (int)(getTotal()/3f*10+0.5)/10f;
}
}
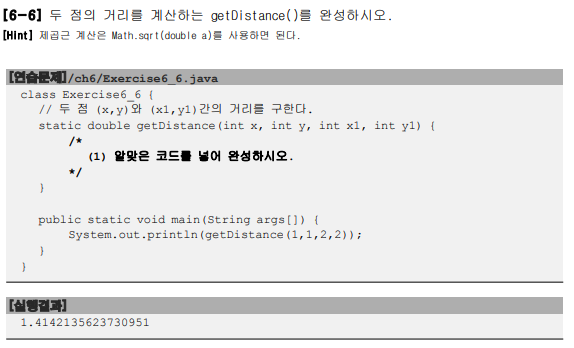
package objectprogm1;
public class Q6_6 {
//두 점 (x,y)와 (x1,y1)간의 거리를 구한다.
static double getDistance(int x, int y, int x1, int y1) {
double squareDistance = (x1-x)*(x1-x)+(y1-y)*(y1-y);
return Math.sqrt(squareDistance);
}
public static void main(String[] args) {
System.out.println(getDistance(1,1,2,2));
}
}
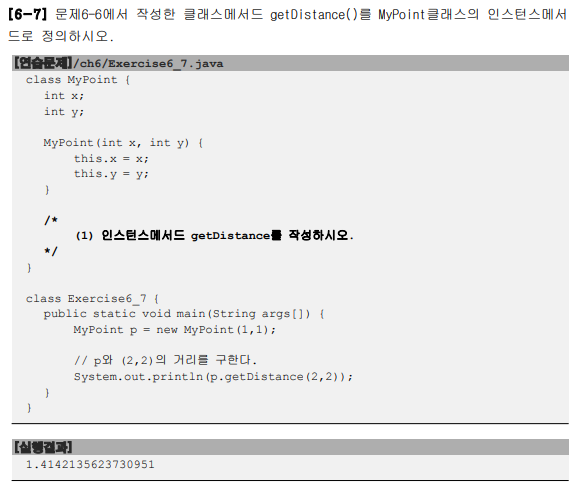
package objectprogm1;
class MyPoint{
int x;
int y;
MyPoint(int x, int y){
this.x = x;
this.y = y;
}
double getDistance(int x1, int y1) {
double squareDistance = (x1-this.x)*(x1-this.x)+(y1-y)*(y1-y);
return Math.sqrt(squareDistance);
}
}
public class Q6_7 {
public static void main(String[] args) {
MyPoint p = new MyPoint(1,1);
System.out.println(p.getDistance(2,2));
}
}
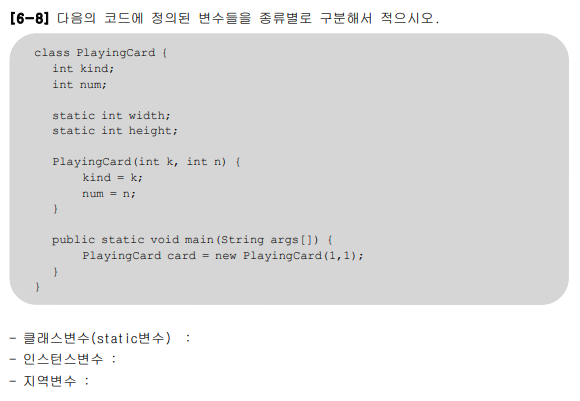
- 클래스 변수 : width, height
- 인스턴스 변수 : kind, num
- 지역변수 : k, n, card, args
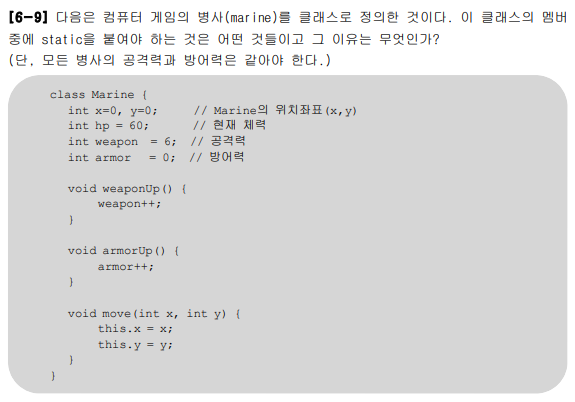
static 변수 : weapon, armor
static 메소드 : weaponUp(), armorUp()
//이유 : 모든 병사의 공격력과 방어력이 같다 = 모든 객체 공통으로 적용되는 것
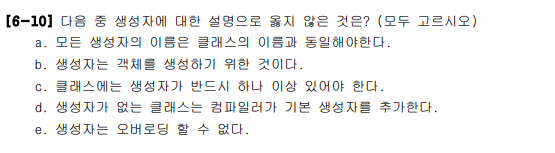
[6-10] b, e
b //초기화를 위한 것
e //오버로딩 가능
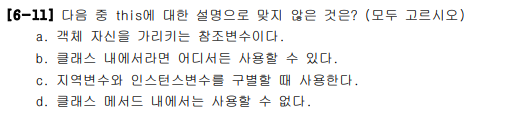
[6-11] b
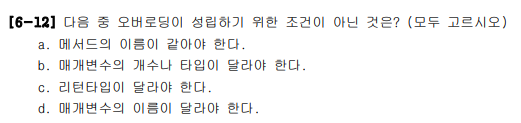
[6-12] c, d
d//이름만 다르고 타입이 갖으면 결국 같은 메소드
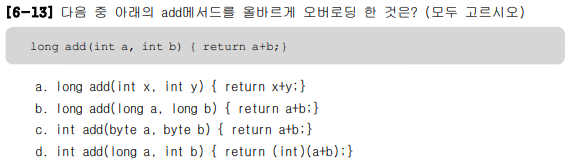
[6-13] b, c, d
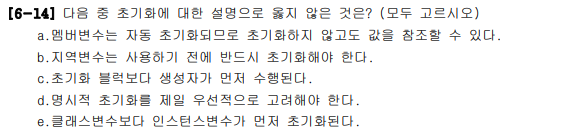
[6-14] c,e
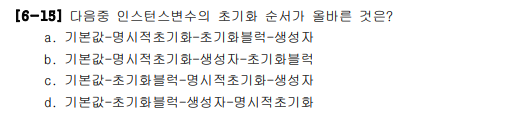
[6-15] a
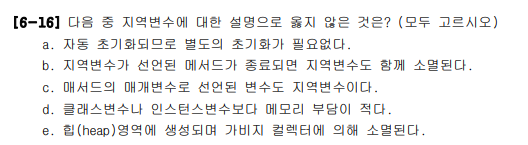
[6-16] a, e
d //지역변수는 자신이 선언된 블럭이나 메소드가 종료되면 소멸되므로 메모리 부담이 적다
e //인스턴스변수를 설명한 것,, 지역변수는 호출스택에 생성된다.
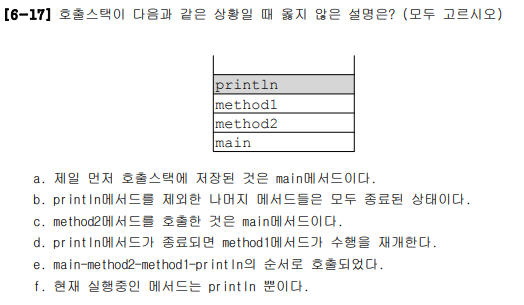
[6-17] b
f //열려있는건 다 열려있지만 실행은 f만 하고 있다.
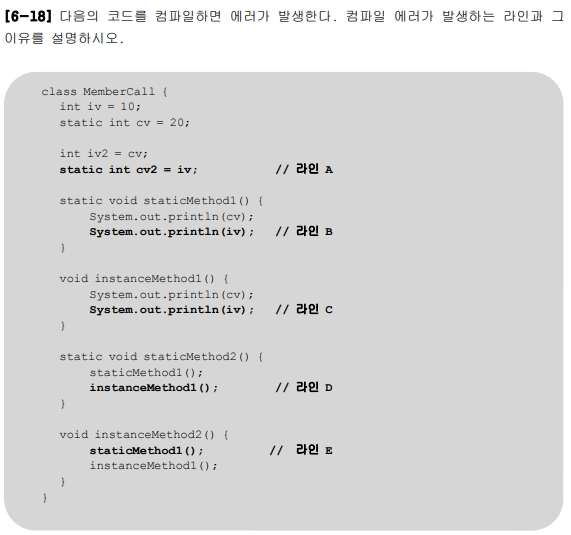
[정답] 라인 A, B, D
[해설]
- 라인 A : static변수의 초기화에 인스턴스 변수를 사용할 수 없다.
꼭 사용해야 한다면 객체를 생성해야 한다.
- 라인 B : static메서드에서는 인스턴스 변수를 사용할 수 없다.
- 라인 C : static메서드에서는 인스턴스메서드를 사용할 수 없다.
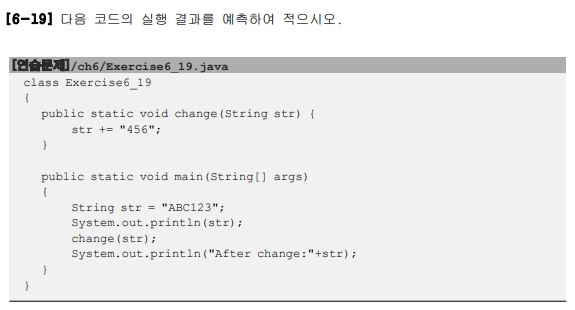
[6-19]
ABC123
After change:ABC123
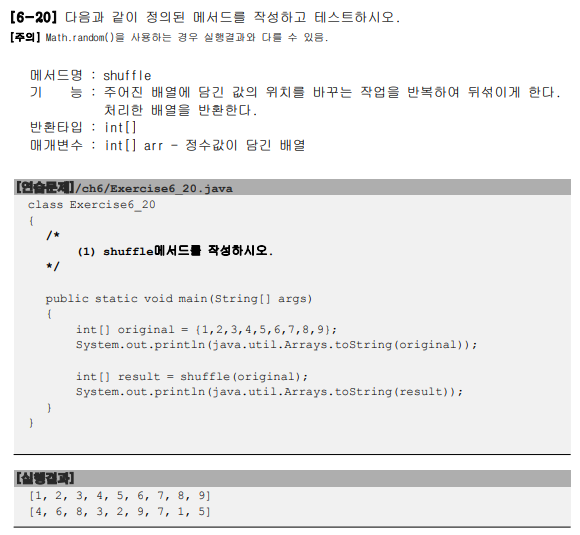
예제 답에서는 arr원본 배열을 shuffle해주었는데, 나는 복사한 배열을 shuffle해서 전달해주었다.
shuffle하는 원리는 동일하다.
package objectprogm1;
public class Q6_20 {
static int[] shuffle(int[] arr) {
//매개변수 유효성 검사
if(arr == null || arr.length == 0) {
return arr;
}
//arr을 복사
int[] sArr = new int[arr.length];
for(int i = 0; i < arr.length; i++) {
sArr[i] = arr[i];
}
//복사본을 shuffle하여 전달
int tmp;
int ran;
for(int i = 0; i < sArr.length; i++) {
ran = (int)(Math.random()*sArr.length);
tmp = sArr[i];
sArr[i] = sArr[ran];
sArr[ran] = tmp;
}
return sArr;
}
public static void main(String[] args) {
int[] original = {1,2,3,4,5,6,7,8,9};
System.out.println(java.util.Arrays.toString(original));
int[] result = shuffle(original);
System.out.println(java.util.Arrays.toString(result));
}
}
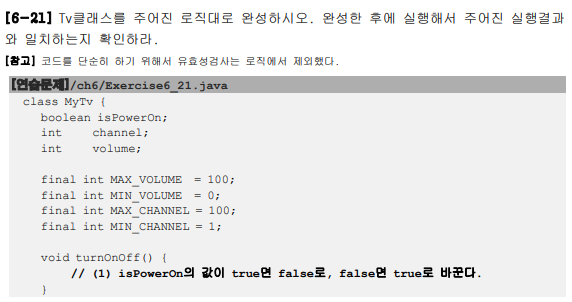
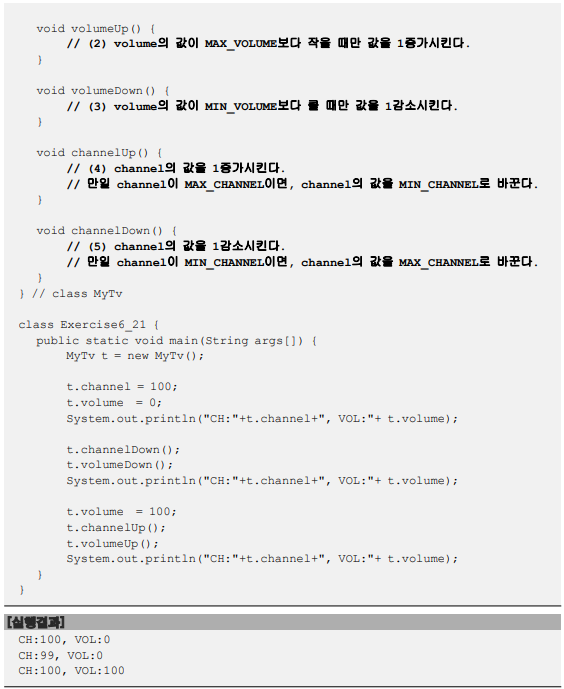
package objectprogm1;
class MyTv{
boolean isPowerOn;
int channel;
int volume;
final int MAX_VOLUME = 100;
final int MIN_VOLUME = 0;
final int MAX_CHANNEL = 100;
final int MIN_CHANNEL = 1;
void turnOnOff() {
isPowerOn = !isPowerOn;
}
void volumeUp() {
this.volume++;
if(volume > MAX_VOLUME)
volume = MAX_VOLUME;
}
void volumeDown() {
this.volume--;
if(volume < MIN_VOLUME)
volume = MIN_VOLUME;
}
void channelUp() {
this.channel++;
if(channel > MAX_CHANNEL)
channel = MAX_CHANNEL;
}
void channelDown() {
this.channel--;
if(channel < MIN_CHANNEL)
channel = MIN_CHANNEL;
}
}//end MyTv
public class Q6_21 {
public static void main(String[] args) {
MyTv t = new MyTv();
t.channel = 100;
t.volume = 0;
System.out.println("CH:"+t.channel+", VOL:"+ t.volume);
t.channelDown();
t.volumeDown();
System.out.println("CH:"+t.channel+", VOL:"+ t.volume);
t.volume = 100;
t.channelUp();
t.volumeUp();
System.out.println("CH:"+t.channel+", VOL:"+ t.volume);
}
}//end class
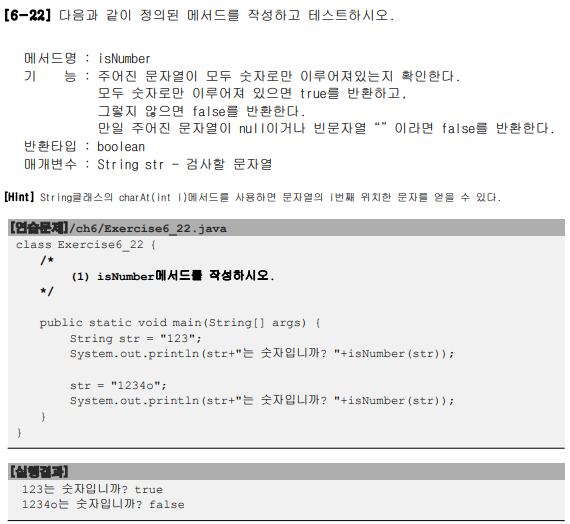
static boolean isNumber(String str) {
//매개변수 유효성 검사
if(str == null || str.equals("")) {
return false;
}
for(int i = 0; i < str.length(); i++) {
int ch = str.charAt(i);
if(!(ch>='0'&&ch<='9')) {
return false;
}
}
return true;
}
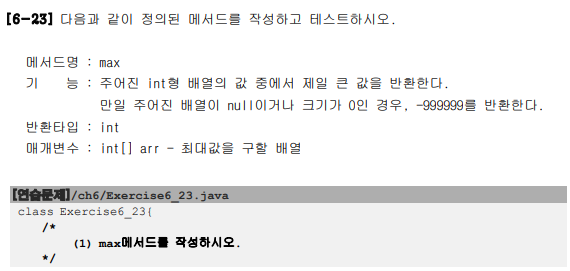
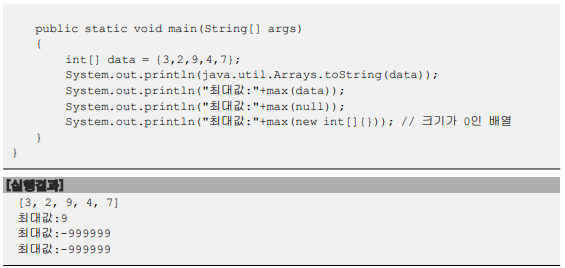
static int max(int[] arr) {
if(arr == null || arr.length == 0) {
return -999999;
}
int max_num = arr[0];
for(int i = 1; i < arr.length; i++) {
if(arr[i]>max_num) {
max_num = arr[i];
}
}
return max_num;
/*
int max_num = 0;
for(int i = 0; i < arr.length-1; i++) {
if(arr[i]>arr[i+1]) {
max_num = arr[i];
}else {
max_num = arr[i+1];
}
}
*/
}

static int abs(int value) {
return value<0? -value : value;
/*
if(value<0) {
return -value;
}else {
return value;
}
*/
}
'Language > Java' 카테고리의 다른 글
[자바API_AWT/Swing] 버튼 이벤트 구현하기 (0) | 2022.03.28 |
---|---|
[자바API_AWT/Swing] 배치관리자와 레이아웃 종류(2)_윈도우창(JFrame) 예제 (0) | 2022.03.27 |
[자바의정석_예제] 조건문과 반복문 (0) | 2022.03.27 |
[자바API_AWT/Swing] 배치관리자와 레이아웃 종류 (0) | 2022.03.26 |
[자바API_AWT/Swing] JFrame 컨테이너로 윈도우창 만들기 (0) | 2022.03.26 |